Hi Charlie,
I now have a fully working date field, which successfully deals with both single events and recurring events.
In summary, what we are doing is creating a cookie via Javascript, which grabs the content of data-mec-cell
from the date clicked on the calendar.
This is then used to create a cookie with the name mec_event_date
. Once the date and event have been selected, the new cookie dynamic content feature grabs the cookie
It then displays the correct date on the single layout page for the event. There is a mechanism built in to deal with the change of month, so the cookie is deleted upon month change and added back when the selected event is chosen.
The time uses your function and DC from post 2 of this thread: {{dc:mec:next_occurrence_start type="date" format="g:ia"}}
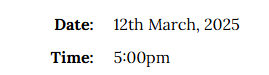
You can see it in action on the single layout you have already seen from earlier secure notes. Here are the steps to get this working, as I am sure you will be able to develop something much neater for a future release:
SINGLE LAYOUT
Following JS added to Global JS:
document.addEventListener("DOMContentLoaded", function () {
function handleDateClick(event) {
let dateElement = event.currentTarget;
let selectedDate = dateElement.getAttribute("data-mec-cell");
// Set the selected date in a cookie
document.cookie = `mec_event_date=${selectedDate}; path=/;`;
}
function attachEventListeners() {
let eventDays = document.querySelectorAll("dt.mec-calendar-day.mec-has-event");
eventDays.forEach(day => {
let eventDate = day.getAttribute("data-mec-cell");
// Remove any existing listener before adding a new one to avoid duplicates
day.removeEventListener("click", handleDateClick);
day.addEventListener("click", handleDateClick);
});
}
function handleMonthChange() {
setTimeout(() => {
setTimeout(() => {
attachEventListeners();
// Delete old cookie when month changes
document.cookie = "mec_event_date=; path=/; expires=Thu, 01 Jan 1970 00:00:00 UTC;";
}, 1000); // Adjust timing if needed
}, 500); // Initial delay after clicking the navigation button
}
function observeMonthChange() {
let monthNavButtons = document.querySelectorAll(".mec-next-month, .mec-previous-month");
monthNavButtons.forEach(button => {
button.removeEventListener("click", handleMonthChange);
button.addEventListener("click", handleMonthChange);
});
}
// General click listener to ensure date selection works after month change
document.addEventListener("click", function (event) {
let dateElement = event.target.closest("dt.mec-calendar-day.mec-has-event");
if (dateElement) {
// Manually trigger handleDateClick to ensure the cookie is set
handleDateClick({ currentTarget: dateElement });
}
});
// Initial setup
attachEventListeners();
observeMonthChange();
});
Date Field To Display The Event Date
{{dc:cookie:get name=βmec_event_dateβ type=βdateβ format="jS F Y}}
Just in case it helps, here is the same Javascript, but with a load of debugging steps included:
document.addEventListener("DOMContentLoaded", function () {
console.log("π Script loaded, initializing MEC event listeners...");
function handleDateClick(event) {
let dateElement = event.currentTarget;
let selectedDate = dateElement.getAttribute("data-mec-cell");
console.log("π
Date clicked with value:", selectedDate);
// Set the selected date in a cookie
document.cookie = `mec_event_date=${selectedDate}; path=/;`;
console.log(`π
Setting mec_event_date to: ${selectedDate}`);
}
function attachEventListeners() {
let eventDays = document.querySelectorAll("dt.mec-calendar-day.mec-has-event");
console.log(`π
Found ${eventDays.length} event dates in this month.`);
eventDays.forEach(day => {
let eventDate = day.getAttribute("data-mec-cell");
console.log("π
Event date found:", eventDate);
// Remove any existing listener before adding a new one to avoid duplicates
day.removeEventListener("click", handleDateClick);
day.addEventListener("click", handleDateClick);
console.log(`β
Listener attached to: ${eventDate}`);
});
console.log("β
Date click listeners successfully attached.");
}
function handleMonthChange() {
console.log("π Month navigation clicked.");
setTimeout(() => {
console.log("π Month changed, waiting for MEC to update...");
setTimeout(() => {
console.log("π
MEC updated, reattaching event listeners...");
attachEventListeners();
// Delete old cookie when month changes
document.cookie = "mec_event_date=; path=/; expires=Thu, 01 Jan 1970 00:00:00 UTC;";
console.log("π« Deleted mec_event_date cookie");
}, 1000); // Adjust timing if needed
}, 500); // Initial delay after clicking the navigation button
}
function observeMonthChange() {
let monthNavButtons = document.querySelectorAll(".mec-next-month, .mec-previous-month");
monthNavButtons.forEach(button => {
button.removeEventListener("click", handleMonthChange);
button.addEventListener("click", handleMonthChange);
});
console.log("π Month navigation listeners attached.");
}
// General click listener to ensure date selection works after month change
document.addEventListener("click", function (event) {
let dateElement = event.target.closest("dt.mec-calendar-day.mec-has-event");
if (dateElement) {
let selectedDate = dateElement.getAttribute("data-mec-cell");
console.log("π
Date clicked from general click listener:", selectedDate);
// Manually trigger handleDateClick to ensure the cookie is set
handleDateClick({ currentTarget: dateElement });
}
});
// Initial setup
attachEventListeners();
observeMonthChange();
});
Thanks,
Christopher